A toggle button allows the user to change a setting between two states.
You can add a basic toggle button to your layout with the ToggleButton
object. Android 4.0 (API level 14) introduces another kind of toggle button called a switch that
provides a slider control, which you can add with a Switch
object.
If you need to change a button's state yourself, you can use the CompoundButton.setChecked()
or
CompoundButton.toggle()
methods.
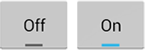
Toggle buttons

Switches (in Android 4.0+)
Responding to Button Presses
To detect when the user activates the button or switch, create an CompoundButton.OnCheckedChangeListener
object and assign it
to the button by calling setOnCheckedChangeListener()
. For example:
ToggleButton toggle = (ToggleButton) findViewById(R.id.togglebutton); toggle.setOnCheckedChangeListener(new CompoundButton.OnCheckedChangeListener() { public void onCheckedChanged(CompoundButton buttonView, boolean isChecked) { if (isChecked) { // The toggle is enabled } else { // The toggle is disabled } } });