This lesson teaches you to
- Set up for Geofence Monitoring
- Create and Add Geofences
- Handle Geofence Transitions
- Stop Geofence Monitoring
You should also read
Try it out
Geofencing combines awareness of the user's current location with awareness of the user's proximity to locations that may be of interest. To mark a location of interest, you specify its latitude and longitude. To adjust the proximity for the location, you add a radius. The latitude, longitude, and radius define a geofence, creating a circular area, or fence, around the location of interest.
You can have multiple active geofences, with a limit of 100 per device user. For each geofence, you can ask Location Services to send you entrance and exit events, or you can specify a duration within the geofence area to wait, or dwell, before triggering an event. You can limit the duration of any geofence by specifying an expiration duration in milliseconds. After the geofence expires, Location Services automatically removes it.
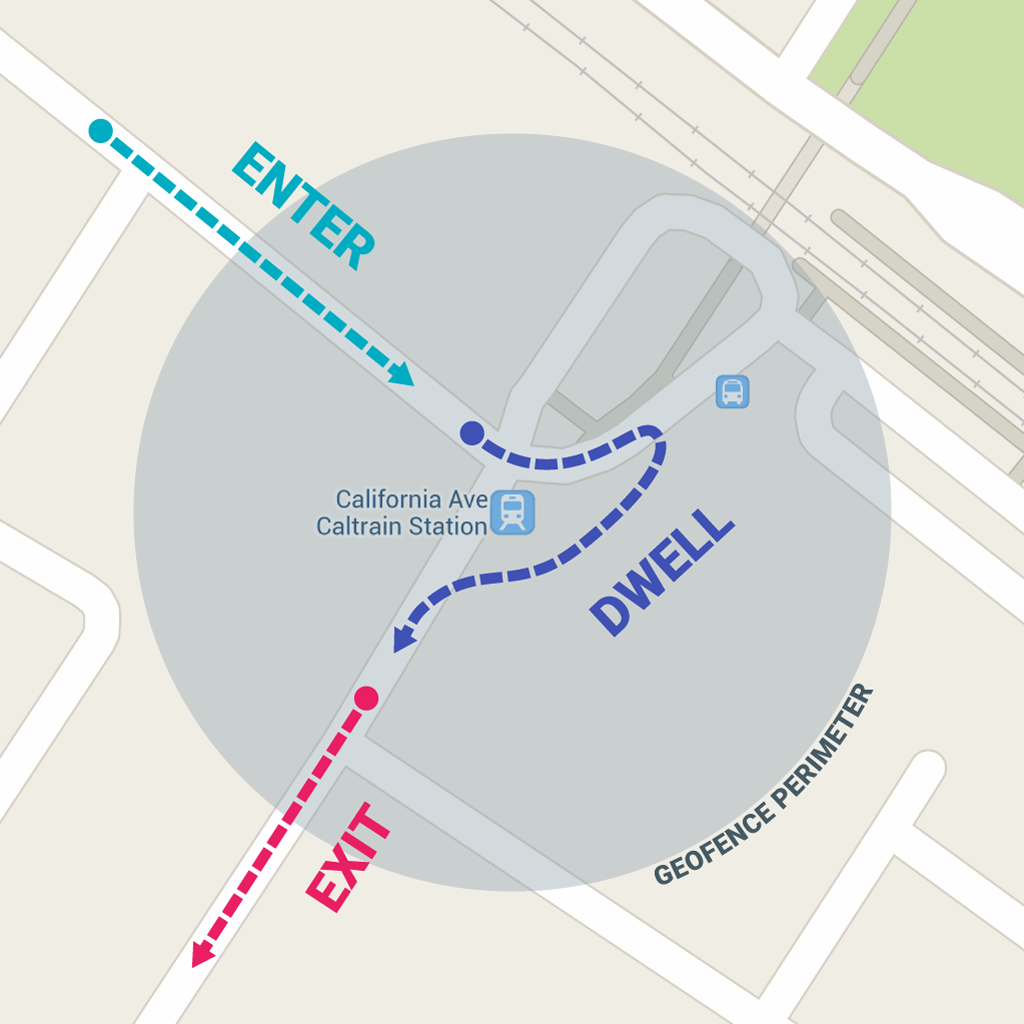
This lesson shows you how to add and remove geofences, and then listen for geofence transitions
using an IntentService
.
Set up for Geofence Monitoring
The first step in requesting geofence monitoring is to request the necessary permission.
To use geofencing, your app must request
ACCESS_FINE_LOCATION
. To request this
permission, add the following element as a child element of the
<manifest>
element in your app manifest:
<uses-permission android:name="android.permission.ACCESS_FINE_LOCATION"/>
If you want to use an IntentService
to listen for geofence transitions,
add an element specifying the service name. This element must be
a child of the
<application>
element:
<application android:allowBackup="true"> ... <service android:name=".GeofenceTransitionsIntentService"/> <application/>
To access the location APIs, you need to create an instance of the Google Play services API client. To learn how to connect your client, see Connect to Google Play Services.
Create and Add Geofences
Your app needs to create and add geofences using the location API's builder class for
creating Geofence objects, and the convenience class for adding them. Also, to handle the
intents sent from Location Services when geofence transitions occur, you can define a
PendingIntent
as shown in this section.
Create geofence objects
First, use Geofence.Builder
to create a geofence, setting the desired radius, duration, and
transition types for the geofence. For example, to populate a list object named
mGeofenceList
:
mGeofenceList.add(new Geofence.Builder() // Set the request ID of the geofence. This is a string to identify this // geofence. .setRequestId(entry.getKey()) .setCircularRegion( entry.getValue().latitude, entry.getValue().longitude, Constants.GEOFENCE_RADIUS_IN_METERS ) .setExpirationDuration(Constants.GEOFENCE_EXPIRATION_IN_MILLISECONDS) .setTransitionTypes(Geofence.GEOFENCE_TRANSITION_ENTER | Geofence.GEOFENCE_TRANSITION_EXIT) .build());
This example pulls data from a constants file. In actual practice, apps might dynamically create geofences based on the user's location.
Specify geofences and initial triggers
The following snippet uses the
GeofencingRequest
class
and its nested
GeofencingRequestBuilder
class to
specify the geofences to monitor and to set how related geofence events are triggered:
private GeofencingRequest getGeofencingRequest() { GeofencingRequest.Builder builder = new GeofencingRequest.Builder(); builder.setInitialTrigger(GeofencingRequest.INITIAL_TRIGGER_ENTER); builder.addGeofences(mGeofenceList); return builder.build(); }
This example shows the use of two geofence triggers. The
GEOFENCE_TRANSITION_ENTER
transition triggers when a device enters a geofence, and the
GEOFENCE_TRANSITION_EXIT
transition triggers when a device exits a geofence. Specifying
INITIAL_TRIGGER_ENTER
tells Location services that
GEOFENCE_TRANSITION_ENTER
should be triggered if the the device is already inside the geofence.
In many cases, it may be preferable to use instead
INITIAL_TRIGGER_DWELL
,
which triggers events only when the user stops for a defined duration within a geofence.
This approach can help reduce "alert spam" resulting from large numbers notifications when a
device briefly enters and exits geofences. Another strategy for getting best results from your
geofences is to set a minimum radius of 100 meters. This helps account for the location accuracy
of typical WiFi networks, and also helps reduce device power consumption.
Define an Intent for geofence transitions
The Intent
sent from Location Services can trigger various actions in
your app, but you should not have it start an activity or fragment, because components
should only become visible in response to a user action. In many cases, an
IntentService
is a good way to handle the intent. An
IntentService
can post a notification, do long-running background work,
send intents to other services, or send a broadcast intent. The following snippet shows how
to define a PendingIntent
that starts an IntentService
:
public class MainActivity extends FragmentActivity { ... private PendingIntent getGeofencePendingIntent() { // Reuse the PendingIntent if we already have it. if (mGeofencePendingIntent != null) { return mGeofencePendingIntent; } Intent intent = new Intent(this, GeofenceTransitionsIntentService.class); // We use FLAG_UPDATE_CURRENT so that we get the same pending intent back when // calling addGeofences() and removeGeofences(). return PendingIntent.getService(this, 0, intent, PendingIntent. FLAG_UPDATE_CURRENT); }
Add geofences
To add geofences, use the
method.
Provide the Google API client, the GeoencingApi.addGeofences()
GeofencingRequest
object, and the PendingIntent
.
The following snippet, which processes the results in
onResult()
, assumes that the main activity implements
ResultCallback
:
public class MainActivity extends FragmentActivity { ... LocationServices.GeofencingApi.addGeofences( mGoogleApiClient, getGeofencingRequest(), getGeofencePendingIntent() ).setResultCallback(this);
Handle Geofence Transitions
When Location Services detects that the user has entered or exited a geofence, it
sends out the Intent
contained in the PendingIntent
you included in the request to add geofences. This Intent
is received
by a service like GeofenceTransitionsIntentService
,
which obtains the geofencing event from the intent, determines the type of Geofence transition(s),
and determines which of the defined geofences was triggered. It then sends a notification as
the output.
The following snippet shows how to define an IntentService
that posts a
notification when a geofence transition occurs. When the user clicks the notification, the
app's main activity appears:
public class GeofenceTransitionsIntentService extends IntentService { ... protected void onHandleIntent(Intent intent) { GeofencingEvent geofencingEvent = GeofencingEvent.fromIntent(intent); if (geofencingEvent.hasError()) { String errorMessage = GeofenceErrorMessages.getErrorString(this, geofencingEvent.getErrorCode()); Log.e(TAG, errorMessage); return; } // Get the transition type. int geofenceTransition = geofencingEvent.getGeofenceTransition(); // Test that the reported transition was of interest. if (geofenceTransition == Geofence.GEOFENCE_TRANSITION_ENTER || geofenceTransition == Geofence.GEOFENCE_TRANSITION_EXIT) { // Get the geofences that were triggered. A single event can trigger // multiple geofences. ListtriggeringGeofences = geofencingEvent.getTriggeringGeofences(); // Get the transition details as a String. String geofenceTransitionDetails = getGeofenceTransitionDetails( this, geofenceTransition, triggeringGeofences ); // Send notification and log the transition details. sendNotification(geofenceTransitionDetails); Log.i(TAG, geofenceTransitionDetails); } else { // Log the error. Log.e(TAG, getString(R.string.geofence_transition_invalid_type, geofenceTransition)); } }
After detecting the transition event via the PendingIntent
,
this IntentService
gets the geofence transition type and tests whether
it is one of the events the app uses to trigger notifications -- either
GEOFENCE_TRANSITION_ENTER
or GEOFENCE_TRANSITION_EXIT
in this case. The service then sends a notification and logs the transition details.
Stop Geofence Monitoring
Stopping geofence monitoring when it is no longer needed or desired can help save battery
power and CPU cycles on the device. You can stop geofence monitoring
in the main activity used to add and remove geofences; removing a geofence stops it
immediately. The API provides methods to
remove geofences either by request IDs, or by removing geofences associated with a given
PendingIntent
.
The following snippet removes geofences by PendingIntent
, stopping all
further notification when the device enters or exits previously added geofences:
LocationServices.GeofencingApi.removeGeofences( mGoogleApiClient, // This is the same pending intent that was used in addGeofences(). getGeofencePendingIntent() ).setResultCallback(this); // Result processed in onResult(). }
You can combine geofencing with other location-aware features, such as periodic location updates. For more information, see the other lessons in this class.