This lesson teaches you to
- Declare Your TV Input Service in the Manifest
- Define Your TV Input Service
- Define Setup and Settings Activities
You should also read
Try It Out
A TV input service represents a media stream source, and lets you present your media content in a linear, broadcast TV fashion as channels and programs. With the TV input service, you can provide parental controls, program guide information, and content ratings. The TV input service works with the Android system TV app, developed for the device and immutable by third-party apps, which ultimately controls and presents content on the TV. See TV Input Framework for more information about the framework architecture and its components.
To develop a TV input service, you implement the following components:
TvInputService
provides long-running and background availability for the TV inputTvInputService.Session
maintains the TV input state and communicates with the hosting appTvContract
describes the channels and programs available to the TV inputTvContract.Channels
represents information about a TV channelTvContract.Programs
describes a TV program with data such as program title and start timeTvTrackInfo
represents an audio, video, or subtitle trackTvContentRating
describes a content rating, allows for custom content rating schemesTvInputManager
provides an API to the system TV app and manages the interaction with TV inputs and apps
Declare Your TV Input Service in the Manifest
Your app manifest must declare your TvInputService
. Within that
declaration, specify the BIND_TV_INPUT
permission to allow the
service to connect the TV input to the system. A system service (TvInputManagerService
)
performs the binding and has that permission. The system TV app sends requests to TV input services
via the TvInputManager
interface. The service declaration must also
include an intent filter that specifies the TvInputService
as the action to perform with the intent. Also within the service declaration, declare the service
meta data in a separate XML resource. The service declaration, the intent filter and the service
meta data are described in the following example.
<service android:name="com.example.sampletvinput.SampleTvInput" android:label="@string/sample_tv_input_label" android:permission="android.permission.BIND_TV_INPUT"> <intent-filter> <action android:name="android.media.tv.TvInputService" /> </intent-filter> <meta-data android:name="android.media.tv.input" android:resource="@xml/sample_tv_input" /> </service>
Define the service meta data in separate XML file, as shown in the following example. The service
meta data must include a setup interface that describes the TV input's initial configuration and
channel scan. Also, the service meta data may (optionally) describe a settings activity for users to
modify the TV input's behavior. The service meta data file is located in the XML resources directory
for your application and must match the name of the resource in the manifest. Using the example
manifest entries above, you would create an XML file in the location
res/xml/sample_tv_input.xml
, with the following contents:
<tv-input xmlns:android="http://schemas.android.com/apk/res/android" <!-- Required: activity for setting up the input --> android:setupActivity="com.example.sampletvinput.SampleTvInputSetupActivity" <!-- Optional: activity for controlling the settings --> android:settingsActivity="com.example.sampletvinput.SampleTvInputSettingsActivity" />
Define Your TV Input Service
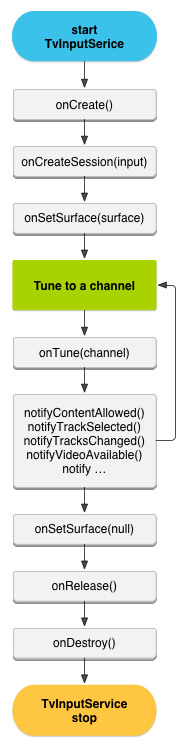
Figure 1.TvInputService lifecycle.
For your service, you extend the TvInputService
class. A
TvInputService
implementation is a
bound service where the system service
(TvInputManagerService
) is the client that binds to it. The service life cycle methods
you need to implement are illustrated in figure 1.
The onCreate()
method initializes and starts the
HandlerThread
which provides a process thread separate from the UI thread to
handle system-driven actions. In the following example, the onCreate()
method initializes the CaptioningManager
and prepares to handle
the ACTION_BLOCKED_RATINGS_CHANGED
and ACTION_PARENTAL_CONTROLS_ENABLED_CHANGED
actions. These
actions describe system intents fired when the user changes the parental control settings, and when
there is a change on the list of blocked ratings.
@Override public void onCreate() { super.onCreate(); mHandlerThread = new HandlerThread(getClass() .getSimpleName()); mHandlerThread.start(); mDbHandler = new Handler(mHandlerThread.getLooper()); mHandler = new Handler(); mCaptioningManager = (CaptioningManager) getSystemService(Context.CAPTIONING_SERVICE); setTheme(android.R.style.Theme_Holo_Light_NoActionBar); mSessions = new ArrayList<BaseTvInputSessionImpl>(); IntentFilter intentFilter = new IntentFilter(); intentFilter.addAction(TvInputManager .ACTION_BLOCKED_RATINGS_CHANGED); intentFilter.addAction(TvInputManager .ACTION_PARENTAL_CONTROLS_ENABLED_CHANGED); registerReceiver(mBroadcastReceiver, intentFilter); }
See
Control Content for more information about working with blocked content and providing
parental control. See TvInputManager
for more system-driven actions that
you may want to handle in your TV input service.
The TvInputService
creates a
TvInputService.Session
that implements Handler.Callback
to handle player state changes. With onSetSurface()
,
the TvInputService.Session
sets the Surface
with the
video content. See Integrate Player with Surface
for more information about working with Surface
to render video.
The TvInputService.Session
handles the
onTune()
event when the user selects a channel, and notifies the system TV app for changes in the content and
content meta data. These notify()
code> methods are described in
Control Content and Handle Track Selection further
in this training.
Define Setup and Settings Activities
The system TV app works with the setup and settings activities you define for your TV input. The setup activity is required and must provide at least one channel record for the system database. The system TV app will invoke the setup activity when it cannot find a channel for the TV input.
The setup activity describes to the system TV app the channels made available through the TV input, as demonstrated in the next lesson, Creating and Updating Channel Data.
The settings activity is optional. You can define a settings activity to turn on parental controls, enable closed captions, set the display attributes, and so forth.