This lesson teaches you to
- Initialize Your Watch Face
- Configure the System UI
- Obtain Information About the Device Screen
- Respond to Changes Between Modes
- Draw Your Watch Face
You should also read
Related Samples
After you have configured your project and added a class that implements the watch face service, you can start writing code to initialize and draw your custom watch face.
This lesson includes examples from the WatchFace sample to show how the system uses the watch face service. Many aspects of the service implementations described here (such as initialization and device features detection) apply to any watch face, so you can reuse some of the code in your own watch faces.
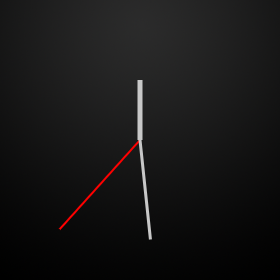
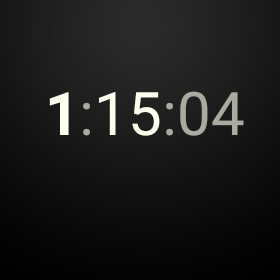
Figure 1. The analog and digital watch faces in the WatchFace sample.
Initialize Your Watch Face
When the system loads your service, you should allocate and initialize most of the resources that your watch face needs, including loading bitmap resources, creating timer objects to run custom animations, configuring paint styles, and performing other computations. You can usually perform these operations only once and reuse their results. This practice improves the performance of your watch face and makes it easier to maintain your code.
To initialize your watch face, follow these steps:
- Declare variables for a custom timer, graphic objects, and other elements.
- Initialize the watch face elements in the
Engine.onCreate()
method. - Initialize the custom timer in the
Engine.onVisibilityChanged()
method.
The following sections describe these steps in detail.
Declare variables
The resources that you intialize when the system loads your service need to be accessible
at different points throughout your implementation, so you can reuse them. You achieve this
by declaring member variables for these resources in your
WatchFaceService.Engine
implementation.
Declare variables for the following elements:
- Graphic objects
- Most watch faces contain at least one bitmap image used as the background of the watch face, as described in Create an Implementation Strategy. You can use additional bitmap images that represent clock hands or other design elements of your watch face.
- Periodic timer
- The system notifies the watch face once a minute when the time changes, but some watch faces run animations at custom time intervals. In these cases, you need to provide a custom timer that ticks with the frequency required to update your watch face.
- Time zone change receiver
- Users can adjust their time zone when they travel, and the system broadcasts this event. Your service implementation must register a broadcast receiver that is notified when the time zone changes and update the time accordingly.
The following snippet shows how to define these variables:
private class Engine extends CanvasWatchFaceService.Engine { static final int MSG_UPDATE_TIME = 0; Calendar mCalendar; // device features boolean mLowBitAmbient; // graphic objects Bitmap mBackgroundBitmap; Bitmap mBackgroundScaledBitmap; Paint mHourPaint; Paint mMinutePaint; ... // handler to update the time once a second in interactive mode final Handler mUpdateTimeHandler = new Handler() { @Override public void handleMessage(Message message) { switch (message.what) { case MSG_UPDATE_TIME: invalidate(); if (shouldTimerBeRunning()) { long timeMs = System.currentTimeMillis(); long delayMs = INTERACTIVE_UPDATE_RATE_MS - (timeMs % INTERACTIVE_UPDATE_RATE_MS); mUpdateTimeHandler .sendEmptyMessageDelayed(MSG_UPDATE_TIME, delayMs); } break; } } }; // receiver to update the time zone final BroadcastReceiver mTimeZoneReceiver = new BroadcastReceiver() { @Override public void onReceive(Context context, Intent intent) { mCalendar.setTimeZone(TimeZone.getDefault()); invalidate(); } }; // service methods (see other sections) ... }
In the example above, the custom timer is implemented as a
Handler
instance that sends and processes delayed messages using the thread's
message queue. For this particular watch face, the custom timer ticks once every second. When the
timer ticks, the handler calls the
invalidate()
method and the system then calls the
onDraw()
method to redraw the watch face.
Initialize watch face elements
After declaring member variables for bitmap resources, paint styles, and other elements that you reuse every time you redraw your watch face, initialize them when the system loads your service. Initializing these elements only once and reusing them improves performance and battery life.
In the
Engine.onCreate()
method, initialize the following elements:
- Load the background image.
- Create styles and colors to draw graphic objects.
- Allocate an object to calculate the time.
- Configure the system UI.
The following snippet shows how to initialize these elements:
@Override public void onCreate(SurfaceHolder holder) { super.onCreate(holder); // configure the system UI (see next section) ... // load the background image Resources resources = AnalogWatchFaceService.this.getResources(); Drawable backgroundDrawable = resources.getDrawable(R.drawable.bg, null); mBackgroundBitmap = ((BitmapDrawable) backgroundDrawable).getBitmap(); // create graphic styles mHourPaint = new Paint(); mHourPaint.setARGB(255, 200, 200, 200); mHourPaint.setStrokeWidth(5.0f); mHourPaint.setAntiAlias(true); mHourPaint.setStrokeCap(Paint.Cap.ROUND); ... // allocate a Calendar to calculate local time using the UTC time and time zone mCalendar = Calendar.getInstance(); }
The background bitmap is loaded only once when the system initializes the watch face. The
graphic styles are instances of the Paint
class. Use these
styles to draw the elements of your watch face inside the
Engine.onDraw()
method, as described in Drawing Your Watch Face.
Initialize the custom timer
As a watch face developer, you decide how often you want to update your watch face by providing a custom timer that ticks with the required frequency while the device is in interactive mode. This enables you to create custom animations and other visual effects.
Note: In ambient mode, the system does not reliably call the custom timer. To update the watch face in ambient mode, see Update the watch face in ambient mode.
An example timer definition from the AnalogWatchFaceService
class that ticks once
every second is shown in Declare variables. In the
Engine.onVisibilityChanged()
method, start the custom timer if these two conditions apply:
- The watch face is visible.
- The device is in interactive mode.
The AnalogWatchFaceService
class schedules the next timer tick if required as
follows:
private void updateTimer() { mUpdateTimeHandler.removeMessages(MSG_UPDATE_TIME); if (shouldTimerBeRunning()) { mUpdateTimeHandler.sendEmptyMessage(MSG_UPDATE_TIME); } } private boolean shouldTimerBeRunning() { return isVisible() && !isInAmbientMode(); }
This custom timer ticks once every second, as described in Declare variables.
In the
onVisibilityChanged()
method, start the timer if required and register the receiver for time zone changes as follows:
@Override public void onVisibilityChanged(boolean visible) { super.onVisibilityChanged(visible); if (visible) { registerReceiver(); // Update time zone in case it changed while we weren't visible. mCalendar.setTimeZone(TimeZone.getDefault()); } else { unregisterReceiver(); } // Whether the timer should be running depends on whether we're visible and // whether we're in ambient mode, so we may need to start or stop the timer updateTimer(); }
When the watch face is visible, the
onVisibilityChanged()
method registers the receiver for time zone changes. If the device is in interactive mode, this
method also starts the custom timer. When the watch face is not visible, this
method stops the custom timer and unregisters the receiver for time zone changes.
The registerReceiver()
and unregisterReceiver()
methods are implemented as
follows:
private void registerReceiver() { if (mRegisteredTimeZoneReceiver) { return; } mRegisteredTimeZoneReceiver = true; IntentFilter filter = new IntentFilter(Intent.ACTION_TIMEZONE_CHANGED); AnalogWatchFaceService.this.registerReceiver(mTimeZoneReceiver, filter); } private void unregisterReceiver() { if (!mRegisteredTimeZoneReceiver) { return; } mRegisteredTimeZoneReceiver = false; AnalogWatchFaceService.this.unregisterReceiver(mTimeZoneReceiver); }
Update the watch face in ambient mode
In ambient mode, the system calls the
Engine.onTimeTick()
method every minute. It is usually sufficient to update your watch face once per minute in this
mode. To update your watch face while in interactive mode, you must provide a custom timer as
described in Initialize the custom timer.
In ambient mode, most watch face implementations simply invalidate the canvas to redraw the watch face in the Engine.onTimeTick() method:
@Override public void onTimeTick() { super.onTimeTick(); invalidate(); }
Configure the System UI
Watch faces should not interfere with system UI elements, as described in Accommodate System UI Elements. If your watch face has a light background or shows information near the bottom of the screen, you may have to configure the size of notification cards or enable background protection.
Android Wear enables you to configure the following aspects of the system UI when your watch face is active:
- Specify how far the first notification card peeks into the screen.
- Specify whether the system draws the time over your watch face.
- Show or hide cards when in ambient mode.
- Protect the system indicators with a solid background around them.
- Specify the positioning of the system indicators.
To configure these aspects of the system UI, create a
WatchFaceStyle
instance and pass it to the
Engine.setWatchFaceStyle()
method.
The AnalogWatchFaceService
class configures the system UI as follows:
@Override public void onCreate(SurfaceHolder holder) { super.onCreate(holder); // configure the system UI setWatchFaceStyle(new WatchFaceStyle.Builder(AnalogWatchFaceService.this) .setCardPeekMode(WatchFaceStyle.PEEK_MODE_SHORT) .setBackgroundVisibility(WatchFaceStyle .BACKGROUND_VISIBILITY_INTERRUPTIVE) .setShowSystemUiTime(false) .build()); ... }
The code snippet above configures peeking cards to be a single line tall, the background of a peeking card to show only briefly and only for interruptive notifications, and the system time not to be shown (since this watch face draws its own time representation).
You can configure the style of the system UI at any point in your watch face implementation. For example, if the user selects a white background, you can add background protection for the system indicators.
For more details about configuring the system UI, see the Wear API reference documentation.
Obtain Information About the Device Screen
The system calls the
Engine.onPropertiesChanged()
method when it determines the properties of the device screen, such as whether the device uses
low-bit ambient mode and whether the screen requires burn-in protection.
The following code snippet shows how to obtain these properties:
@Override public void onPropertiesChanged(Bundle properties) { super.onPropertiesChanged(properties); mLowBitAmbient = properties.getBoolean(PROPERTY_LOW_BIT_AMBIENT, false); mBurnInProtection = properties.getBoolean(PROPERTY_BURN_IN_PROTECTION, false); }
You should take these device properties into account when drawing your watch face:
- For devices that use low-bit ambient mode, the screen supports fewer bits for each color in ambient mode, so you should disable anti-aliasing and bitmap filtering when the device switches to ambient mode.
- For devices that require burn-in protection, avoid using large blocks of white pixels in ambient mode and do not place content within 10 pixels of the edge of the screen, since the system shifts the content periodically to avoid pixel burn-in.
For more information about low-bit ambient mode and burn-in protection, see Optimize for Special Screens. For more information on how to disable bitmap filtering, see Bitmap Filtering.
Respond to Changes Between Modes
When the device switches between ambient and interactive modes, the system calls the
Engine.onAmbientModeChanged()
method. Your service implementation should make any necessary adjustments to switch between modes
and then call the
invalidate()
method for the system to redraw the watch face.
The following snippet shows how to implement this method:
@Override public void onAmbientModeChanged(boolean inAmbientMode) { super.onAmbientModeChanged(inAmbientMode); if (mLowBitAmbient) { boolean antiAlias = !inAmbientMode; mHourPaint.setAntiAlias(antiAlias); mMinutePaint.setAntiAlias(antiAlias); mSecondPaint.setAntiAlias(antiAlias); mTickPaint.setAntiAlias(antiAlias); } invalidate(); updateTimer(); }
This example makes adjustments to some graphic styles and invalidates the canvas so the system can redraw the watch face.
Draw Your Watch Face
To draw a custom watch face, the system calls the
Engine.onDraw()
method with a Canvas
instance and the bounds in which you should draw your
watch face. The bounds take into account any inset areas, such as the "chin" on the bottom of some
round devices. You can use this canvas to draw your watch face directly as follows:
- Override the
onSurfaceChanged()
method to scale your background to fit the device any time the view changes.@Override public void onSurfaceChanged( SurfaceHolder holder, int format, int width, int height) { if (mBackgroundScaledBitmap == null || mBackgroundScaledBitmap.getWidth() != width || mBackgroundScaledBitmap.getHeight() != height) { mBackgroundScaledBitmap = Bitmap.createScaledBitmap(mBackgroundBitmap, width, height, true /* filter */); } super.onSurfaceChanged(holder, format, width, height); }
- Check whether the device is in ambient mode or interactive mode.
- Perform any required graphic computations.
- Draw your background bitmap on the canvas.
- Use the methods in the
Canvas
class to draw your watch face.
The following snippet shows how to implement the
onDraw()
method:
@Override public void onDraw(Canvas canvas, Rect bounds) { // Update the time mCalendar.setTimeInMillis(System.currentTimeMillis()); // Constant to help calculate clock hand rotations final float TWO_PI = (float) Math.PI * 2f; int width = bounds.width(); int height = bounds.height(); canvas.drawBitmap(mBackgroundScaledBitmap, 0, 0, null); // Find the center. Ignore the window insets so that, on round watches // with a "chin", the watch face is centered on the entire screen, not // just the usable portion. float centerX = width / 2f; float centerY = height / 2f; // Compute rotations and lengths for the clock hands. float seconds = mCalendar.get(Calendar.SECOND) + mCalendar.get(Calendar.MILLISECOND) / 1000f; float secRot = seconds / 60f * TWO_PI; float minutes = mCalendar.get(Calendar.MINUTE) + seconds / 60f; float minRot = minutes / 60f * TWO_PI; float hours = mCalendar.get(Calendar.HOUR) + minutes / 60f; float hrRot = hours / 12f * TWO_PI; float secLength = centerX - 20; float minLength = centerX - 40; float hrLength = centerX - 80; // Only draw the second hand in interactive mode. if (!isInAmbientMode()) { float secX = (float) Math.sin(secRot) * secLength; float secY = (float) -Math.cos(secRot) * secLength; canvas.drawLine(centerX, centerY, centerX + secX, centerY + secY, mSecondPaint); } // Draw the minute and hour hands. float minX = (float) Math.sin(minRot) * minLength; float minY = (float) -Math.cos(minRot) * minLength; canvas.drawLine(centerX, centerY, centerX + minX, centerY + minY, mMinutePaint); float hrX = (float) Math.sin(hrRot) * hrLength; float hrY = (float) -Math.cos(hrRot) * hrLength; canvas.drawLine(centerX, centerY, centerX + hrX, centerY + hrY, mHourPaint); }
This method computes the required positions for the clock hands based on the current time
and draws them on top of the background bitmap using the graphic styles initialized in the
onCreate()
method. The second hand is only drawn in interactive mode, not in ambient mode.
For more information about drawing on a Canvas instance, see Canvas and Drawables.
The WatchFace sample includes additional
watch faces that you can refer to as examples of how to implement the
onDraw()
method.