This lesson teaches you to


When creating notifications for a handheld device, you should always aggregate similar notifications into a single summary notification. For example, if your app creates notifications for received messages, you should not show more than one notification on a handheld device—when more than one is message is received, use a single notification to provide a summary such as "2 new messages."
However, a summary notification is less useful on an Android wearable because users
are not able to read details from each message on the wearable (they must open your app on the
handheld to view more information). So for the wearable device, you should
group all the notifications together in a stack. The stack of notifications appears as a single
card, which users can expand to view the details from each notification separately. The new
setGroup()
method makes this
possible while allowing you to still provide only one summary notification on the handheld device.
Add Each Notification to a Group
To create a stack, call setGroup()
for each notification you want in the stack and specify a
group key. Then call notify()
to send it to the wearable.
final static String GROUP_KEY_EMAILS = "group_key_emails"; // Build the notification, setting the group appropriately Notification notif = new NotificationCompat.Builder(mContext) .setContentTitle("New mail from " + sender1) .setContentText(subject1) .setSmallIcon(R.drawable.new_mail) .setGroup(GROUP_KEY_EMAILS) .build(); // Issue the notification NotificationManagerCompat notificationManager = NotificationManagerCompat.from(this); notificationManager.notify(notificationId1, notif);
Later on, when you create another notification, specify
the same group key. When you call
notify()
,
this notification appears in the same stack as the previous notification,
instead of as a new card:
Notification notif2 = new NotificationCompat.Builder(mContext) .setContentTitle("New mail from " + sender2) .setContentText(subject2) .setSmallIcon(R.drawable.new_mail) .setGroup(GROUP_KEY_EMAILS) .build(); notificationManager.notify(notificationId2, notif2);
By default, notifications appear in the order in which you added them, with the most recent
notification visible at the top. You can order notifications in another fashion by calling
setSortKey()
.
Add a Summary Notification
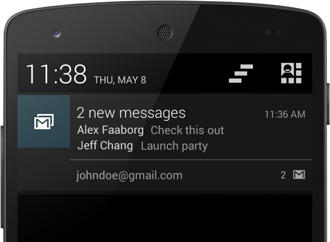
It's important that you still provide a summary notification that appears on handheld devices.
So in addition to adding each unique notification to the same stack group, also add a summary
notification and call setGroupSummary()
on the summary notification.
This notification does not appear in your stack of notifications on the wearable, but it appears as the only notification on the handheld device.
Bitmap largeIcon = BitmapFactory.decodeResource(getResources(), R.drawable.ic_large_icon); // Create an InboxStyle notification Notification summaryNotification = new NotificationCompat.Builder(mContext) .setContentTitle("2 new messages") .setSmallIcon(R.drawable.ic_small_icon) .setLargeIcon(largeIcon) .setStyle(new NotificationCompat.InboxStyle() .addLine("Alex Faaborg Check this out") .addLine("Jeff Chang Launch Party") .setBigContentTitle("2 new messages") .setSummaryText("johndoe@gmail.com")) .setGroup(GROUP_KEY_EMAILS) .setGroupSummary(true) .build(); notificationManager.notify(notificationId3, summaryNotification);
This notification uses NotificationCompat.InboxStyle
,
which gives you an easy way to create notifications for email or messaging apps.
You can use this style, another one defined in NotificationCompat
,
or no style for the summary notification.
Tip: To style the text like in the example screenshot, see Styling with HTML markup and Styling with Spannables.
Summary notifications can also affect notifications on wearables without being displayed on them.
When creating a summary notification, you can use the
NotificationCompat.WearableExtender
class and call
setBackground()
or
addAction()
to set
a background image or an action that applies to the entire stack on the wearable. For instance,
to set the background for an entire stack of notifications:
Bitmap background = BitmapFactory.decodeResource(getResources(), R.drawable.ic_background); NotificationCompat.WearableExtender wearableExtender = new NotificationCompat.WearableExtender() .setBackground(background); // Create an InboxStyle notification Notification summaryNotificationWithBackground = new NotificationCompat.Builder(mContext) .setContentTitle("2 new messages") ... .extend(wearableExtender) .setGroup(GROUP_KEY_EMAILS) .setGroupSummary(true) .build();